Amplitude - Add feature flag changes to your charts
Overview
There are two available integration opportunities between ConfigCat and Amplitude:
- Monitoring your feature flag change events in Amplitude with Annotations
- Sending feature flag evaluation analytics to Amplitude Experiments
Monitoring your feature flag change events in Amplitude with Annotations
Every feature flag change in ConfigCat is annotated on the Amplitude charts as a vertical line and some details are added automatically about the change.
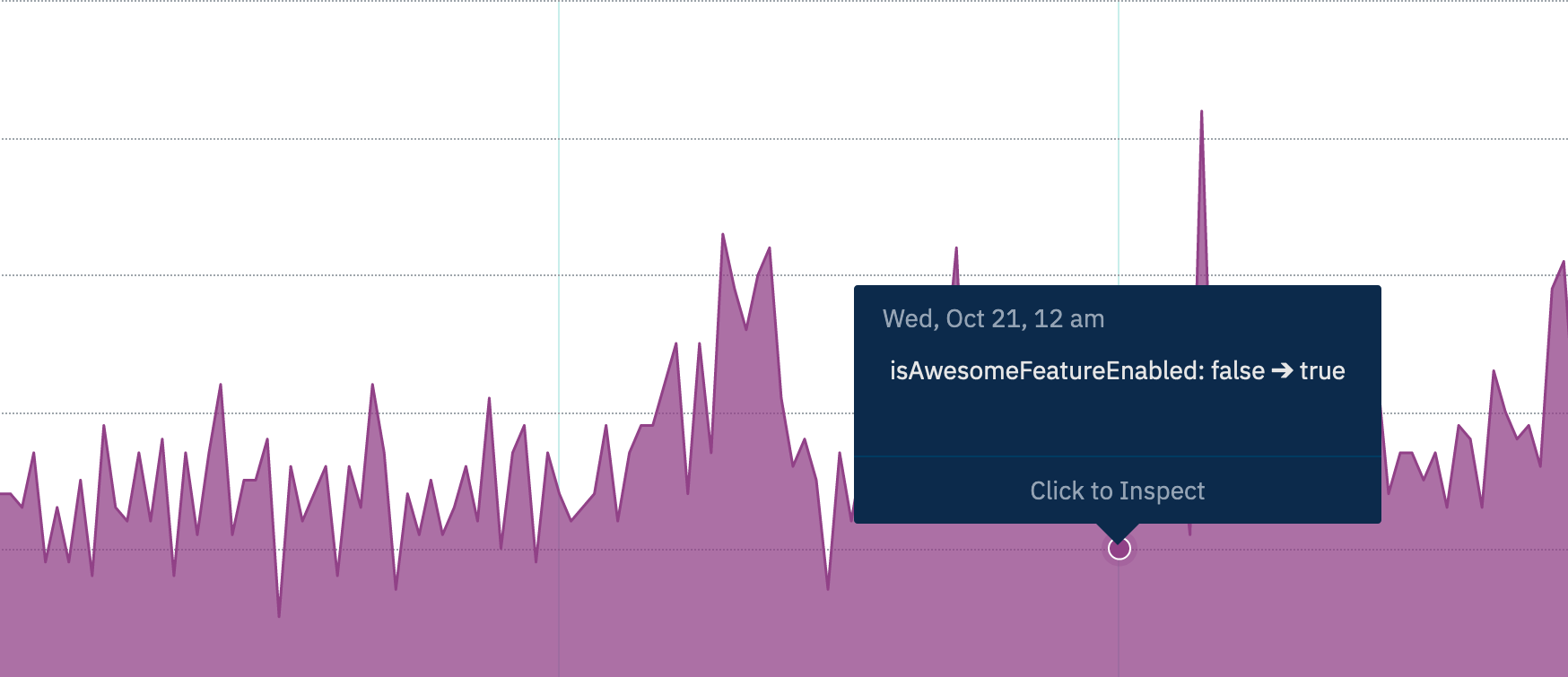
Installation
- Have an Amplitude subscription.
- Get an Amplitude API Key and Secret Key.
- Open the integrations tab on ConfigCat Dashboard.
- Click on Amplitude's CONNECT button and set your Amplitude API key and Secret key.
- You're all set. Go ahead and make some changes on your feature flags, then check your charts in Amplitude.
Un-installation
- Open the integrations tab on ConfigCat Dashboard.
- Click on Amplitude's DISCONNECT button.
Chart Annotation
Every annotation sent to Amplitude by ConfigCat has:
- Name: A brief summary of the change.
- Description: A direct link to the Product/Config/Environment of the feature flag in ConfigCat.
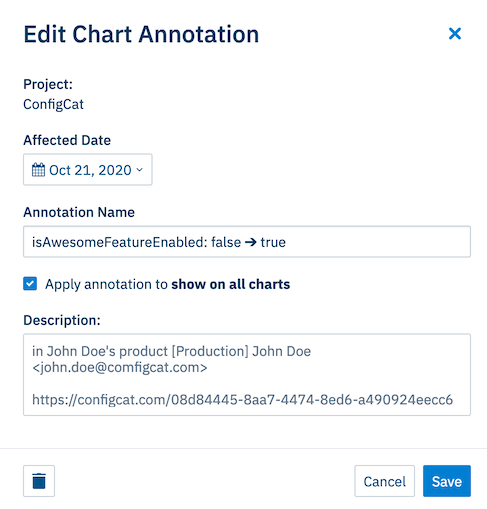
Sending feature flag evaluation analytics to Amplitude Experiments
Ensures that feature flag evaluations are logged into Amplitude Experiments. With this integration, you can have advanced analytics about your feature flag usages, A/B test results.
Setup
- Install SDKs: Add both the ConfigCat SDK and Amplitude SDK to your application.
- Configure SDKs:
- ConfigCat SDK: Initialize with your ConfigCat SDK key.
- Amplitude SDK: Set up with your Amplitude ApiKey.
- Integrate Feature Flag Evaluations:
- During the initialization of the ConfigCat SDK, subscribe to the
flagEvaluated
hook. - Send feature flag evaluation data to Amplitude using the
$exposure
event name. Include the following parameters:flag_key
: the feature flag's key.variant
: the evaluated feature flag's value or variation IDvariation_id
(optional): the evaluated feature flag's variation ID
- You can use the Identify API in Amplitude to enrich all your events with feature flag metadata. This way you can easily group/filter your existing Amplitude events by feature flag evaluations.
- During the initialization of the ConfigCat SDK, subscribe to the
Code samples:
- JavaScript, Node, SSR
- React
- Python
- Go
- Java
- Android
- Swift (iOS)
- Other languages
const configCatClient = configcat.getClient("#YOUR_SDK_KEY", PollingMode.AutoPoll, {
setupHooks: (hooks) =>
hooks.on('flagEvaluated', evaluationDetails => {
// Send an `$exposure` event.
amplitude.track('$exposure',
{
'flag_key': evaluationDetails.key,
'variant': evaluationDetails.value,
'variation_id': evaluationDetails.variationId
});
// Use the identify API.
const identifyEvent = new amplitude.Identify();
identifyEvent.set("configcat_" + evaluationDetails.key, evaluationDetails.value);
amplitude.identify(identifyEvent);
}),
});
<ConfigCatProvider
sdkKey="#YOUR_SDK_KEY"
pollingMode={PollingMode.AutoPoll}
options={{
setupHooks: (hooks) =>
hooks.on('flagEvaluated', evaluationDetails => {
// Send an `$exposure` event.
amplitude.track('$exposure',
{
'flag_key': evaluationDetails.key,
'variant': evaluationDetails.value,
'variation_id': evaluationDetails.variationId
});
// Use the identify API.
const identifyEvent = new amplitude.Identify();
identifyEvent.set("configcat_" + evaluationDetails.key, evaluationDetails.value);
amplitude.identify(identifyEvent);
}),
}}
>
</ConfigCatProvider>
def on_flag_evaluated(evaluation_details):
# Send an `$exposure` event.
amplitude.track(
BaseEvent(
event_type="$exposure",
user_id=evaluation_details.user.get_identifier(),
event_properties={
"flag_key": evaluation_details.key,
"variant": evaluation_details.value,
"variation_id": evaluation_details.variation_id
}
))
# Use the identify API.
identify_obj=Identify()
identify_obj.set(f'configcat_{evaluationDetails.key}', evaluation_details.value)
amplitude.identify(identify_obj, EventOptions(user_id=evaluation_details.user.get_identifier()))
pass
client = configcatclient.get('#YOUR-SDK-KEY#',
ConfigCatOptions(
hooks=Hooks(on_flag_evaluated=on_flag_evaluated)
)
)
client := configcat.NewCustomClient(configcat.Config{SDKKey: "#YOUR-SDK-KEY#",
Hooks: &configcat.Hooks{OnFlagEvaluated: func(details *configcat.EvaluationDetails) {
// Send an `$exposure` event.
amplitude.Track(amplitude.Event{
UserID: details.Data.User.(*configcat.UserData).Identifier,
EventType: "$exposure",
EventProperties: map[string]interface{}{
"flag_key": details.Data.Key,
"variant": details.Value,
"variation_id": details.Data.VariationID,
},
})
// Use the identify API.
identifyObj := amplitude.Identify{}
identifyObj.Set("configcat_" + details.Data.Key, details.Value)
amplitude.Identify(identifyObj, amplitude.EventOptions{UserID: details.Data.User.(*configcat.UserData).Identifier})
}}})
ConfigCatClient client = ConfigCatClient.get("#YOUR-SDK-KEY#", options -> {
options.hooks().addOnFlagEvaluated(details -> {
// Send an `$exposure` event.
JSONObject eventProps = new JSONObject();
eventProps.put("flag_key", details.getKey());
eventProps.put("variant", details.getValue());
eventProps.put("variation_id", details.getVariationId());
Event event = new Event("$exposure", details.getUser().getIdentifier());
amplitude.logEvent(event);
// Use the identify API.
JSONObject userProps = new JSONObject();
userProps.put("configcat_" + details.getKey(), details.getValue());
Event updateUser = new Event("$identify", details.getUser().getIdentifier());
updateUser.userProperties = userProps;
amplitude.logEvent(event);
});
});
ConfigCatClient client = ConfigCatClient.get("#YOUR-SDK-KEY#", options -> {
options.hooks().addOnFlagEvaluated(details -> {
// Send an `$exposure` event.
amplitude.track(
"$exposure",
mutableMapOf<String, Any?>(
"flag_key" to details.getKey(),
"variant" to details.getValue(),
"variation_id" to details.getVariationId()
))
// Use the identify API.
val identify = Identify()
identify.set("configcat_" + details.getKey(), details.getValue())
});
});
let client = ConfigCatClient.get(sdkKey: "#YOUR-SDK-KEY#") { options in
options.hooks.addOnFlagEvaluated { details in
// Send an `$exposure` event.
let event = BaseEvent(
eventType: "$exposure",
eventProperties: [
"flag_key": details.key,
"variant": details.value,
"variation_id": details.variationId ?? ""
]
)
// Use the identify API.
let identify = Identify()
identify.set(property: "configcat_" + details.key, value: details.value)
amplitude.identify(identify: identify)
}
}
While our documentation primarily provides code examples for languages that Amplitude natively supports and has an official SDK, you can integrate with other languages by sending an event to Amplitude with a third-party SDK or with using the Amplitude's Upload request API.
- Subscribe to the FlagEvaluated hook in the ConfigCat SDK.
- Send an event to Amplitude using the
$exposure
event name. Include the following event properties:flag_key
: the feature flag's key from the FlagEvaluated hook's EvaluationDetailsvariant
: the evaluated feature flag's value or the variationId from the FlagEvaluated hook's EvaluationDetailsvariation_id
: the evaluated feature flag's value or the variationId from the FlagEvaluated hook's EvaluationDetailsuser_id
(optional): in case you are using the tracking in a backend component or you don't identify all your event sendings to Amplitude with user details, you have to send theuser_id
property as well to identify your user. You can use the User object's Identifier property from the FlagEvaluated hook or a value that best describes your user.
For Text feature flags with lengthy values (e.g., JSON), send the variationId
instead of the value
as the variant
to Amplitude.
The variationId
is a hashed version of the feature flag value, accessible on the ConfigCat Dashboard by enabling the Show VariationIDs to support A/B testing setting. Learn more here.
- Deploy your application and wait for feature flag evaluations to happen so Experiments in Amplitude could be populated.
Usage with Experiments
Check your Experiments page in Amplitude and select your feature flag as the Experiment.
Usage with custom chart
If you don't have access to the Experiments feature in Amplitude, you can create a custom chart based on the Exposure
event.
You can filter for your feature flag keys with the Flag Key
property and visualize the different variants by using the Variant
property as a Breakdown. Example:
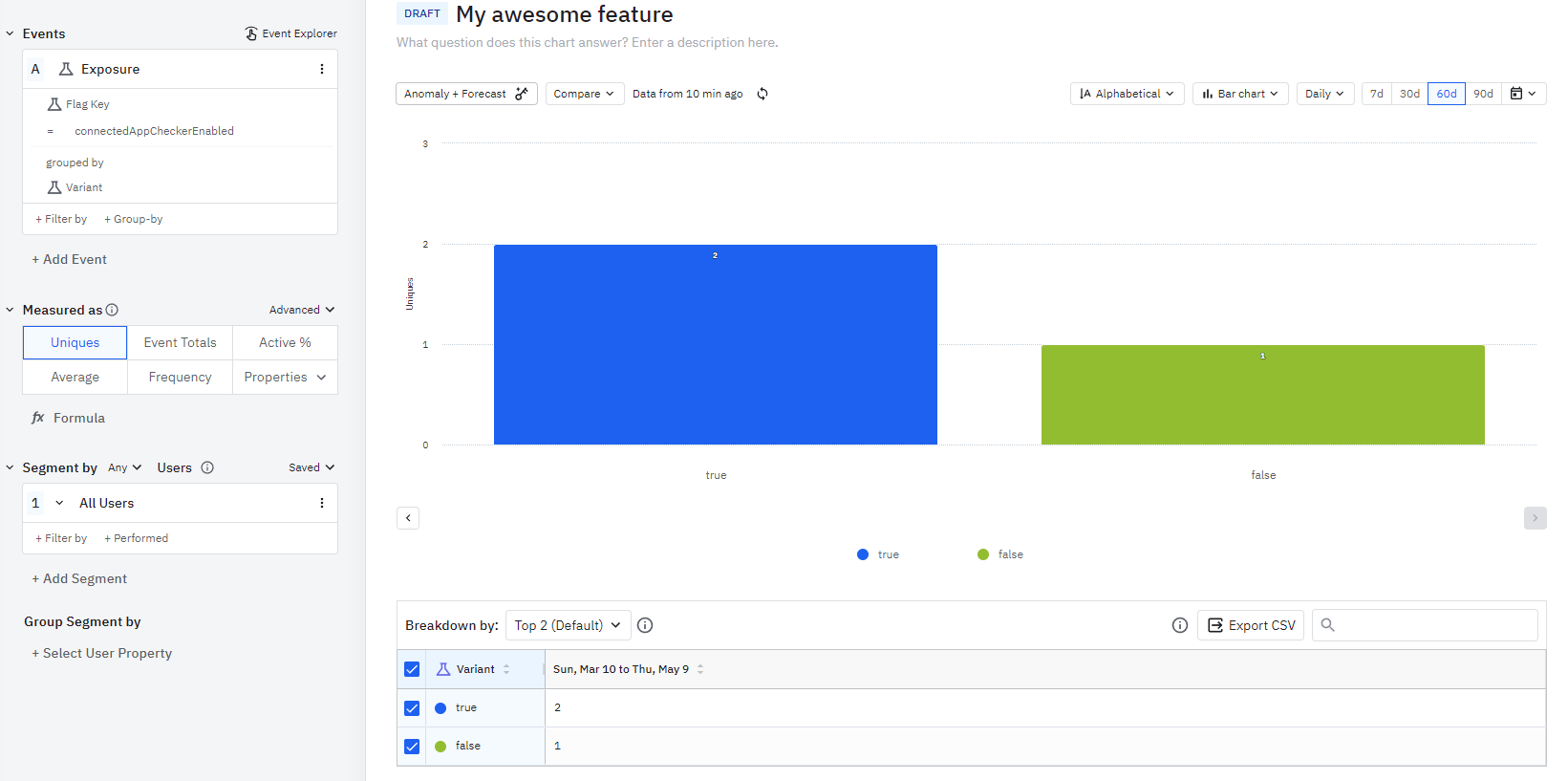
Usage with enriched user properties for your custom events.
If you use the Identify API approach, you'll be able to use the feature flag evaluation data in your current reports. You can Group Segments by your feature flag evaluations:
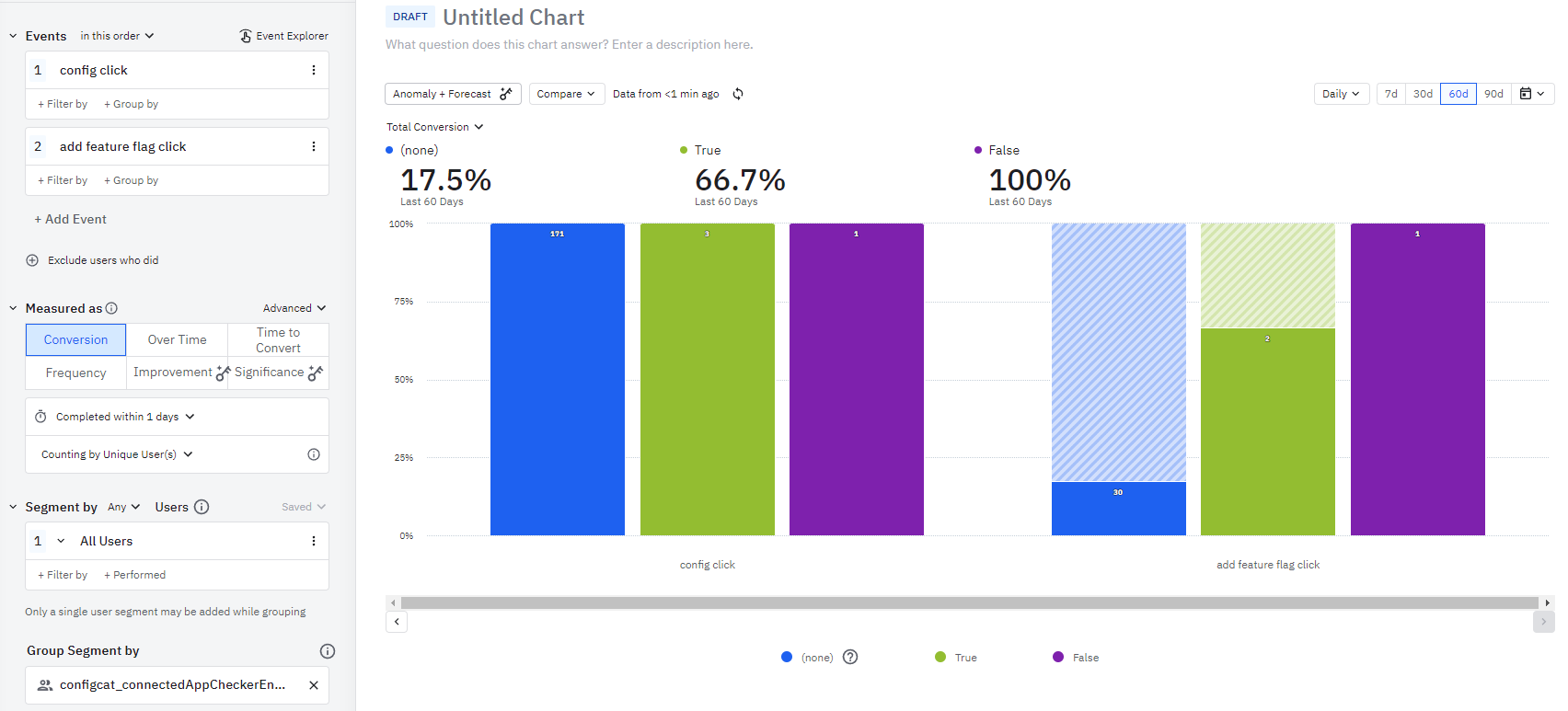