Using Twilio and ConfigCat to Understand Your Business
Understanding how users interact with your product's features is essential for delivering optimal user experiences. Twilio Segment and ConfigCat are two great tools that work well together to help you get valuable insights into how users engage with your app. Let's take a look at how it works!
In the following sections, we'll discuss the practical applications, outline the benefits, and walk through an example of how to set up the integration in a React.js app.
Using Twilio Segment with ConfigCat
With the integration of Twilio Segment and ConfigCat, you can capture real-time events related to your feature flags. This means you’ll know when changes happen in ConfigCat and how users respond to those changes in your app. You can then send this information to various places like analytics tools, marketing platforms, or data warehouses.
What Information Is Sent to Twilio Segment?
-
Feature flag evaluation events: ConfigCat sends details such as the feature flag's key, the evaluated value, the variation ID, and user information used during the evaluation. This event occurs when a feature flag is evaluated in the application.
-
Feature flag change events: When a feature flag is modified in the ConfigCat dashboard, details like the product ID, config name, environment name, and the user who made the changes are sent to Twilio Segment.
-
User interactions: When a user interacts with the software, such as by clicking on buttons, these interactions can also be tracked.
These events offer businesses visibility into feature usage and modification, which can be critical in maintaining an efficient and well-performing software product.
How Event Tracking Enhances Business Operations
Tracking feature flag change events provides businesses with immediate feedback on feature updates. Knowing exactly when and how features are modified can improve the user experience. For example, problematic features can be quickly identified and rolled back if an issue arises, minimizing negative user impact. Using data collected through Twilio Segment, businesses can conduct A/B tests to track events and analyze which variation of a feature performs better.
Example: Increasing Sign-Up Conversions
Suppose a business wants to improve the conversion rate of its signup form. This can be done by creating two versions (Form A and Form B) and using ConfigCat's feature flag service to control which version users see. By capturing events from both forms with Twilio Segment, the business can track whether users complete the signup process. The data can then be analyzed on a platform like Amplitude to see which form has a higher conversion rate. You can read more about the optimization and analytics of conversion events here.
Let's examine a demo of how businesses can gain insight into their products' performance using real-time feature flag evaluations.
Demo: Using ConfigCat and Twilio Segment Integration in a React.js App
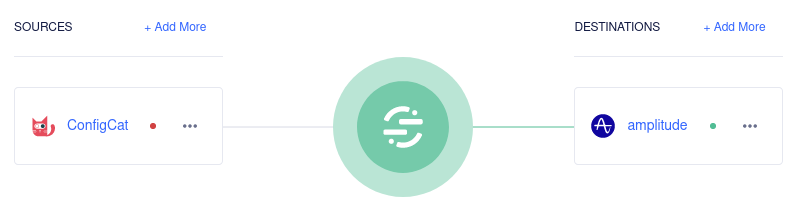
In the following section, we'll explore how this integration can track feature flag evaluation events and user interactions in a React.js app.
In addition to React.js, you can integrate Twilio Segment and ConfigCat with other programming languages. To learn more, click here.
Prerequisites
To follow along, you'll need:
- A Twilio Segment account
- A ConfigCat account
- An Amplitude account
- A React.js app with the ConfigCat React SDK installed.
If you need help setting up your React.js App with ConfigCat, feel free to explore the React.js SDK documentation or check out our blog post on using feature flags in React.
1. Set Up Twilio Segment Analytics in the React.js App
Install the Segment Analytics SDK in your React.js app and initialize it in your App.js
file:
npm install @segment/analytics-next
import { AnalyticsBrowser } from '@segment/analytics-next';
const segmentAnalytics = AnalyticsBrowser.load({
writeKey: 'YOUR_SEGMENT_WRITE_KEY',
});
2. Add Middleware to Segment
Create a Source Middleware that sends a payload containing the integration information to Twilio Segment.
segmentAnalytics.addSourceMiddleware(({ payload, next }) => {
if (payload.obj.context) {
payload.obj.context.integration = payload.obj.context.integration || {};
payload.obj.context.integration['name'] = 'configcat';
payload.obj.context.integration['version'] = '1.0.0';
} else {
payload.obj.context = {
integration: {
name: 'configcat',
version: '1.0.0',
},
};
}
next(payload);
});
3. Configure ConfigCat to Send Events to Twilio Segment
To add ConfigCat as a Source in Segment, you'll need your Segment Write Key
. This can be done in a few easy steps:
-
Log in to your Segment account and navigate to the Sources catalog page.
-
Search for "ConfigCat", select it, and click
Add Source
.
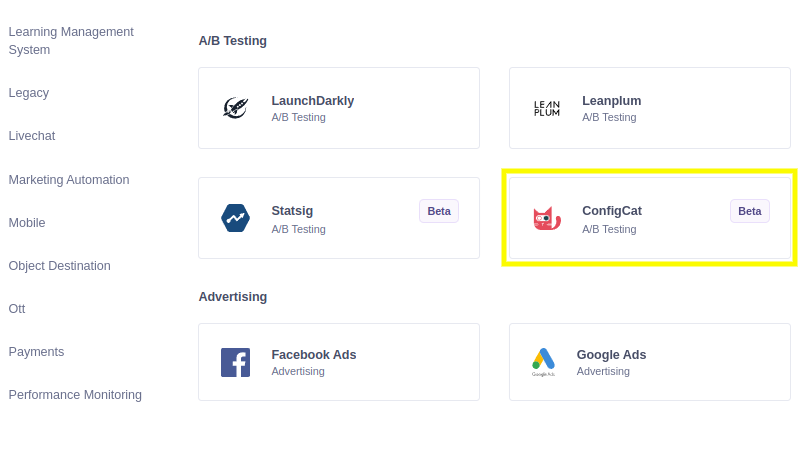
- Give the source a name and click
Add Source
to save your settings.
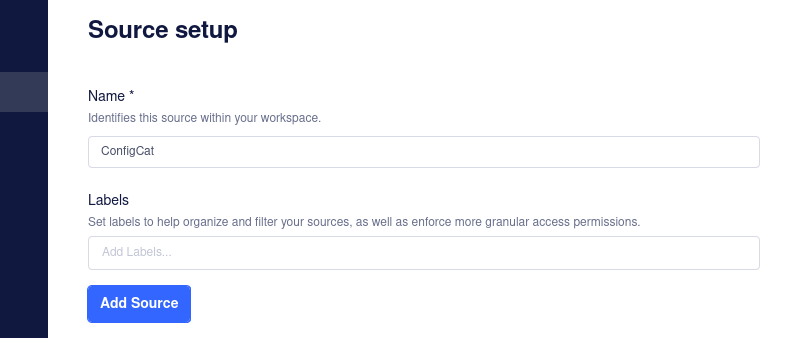
- Copy the Write Key from the Segment.
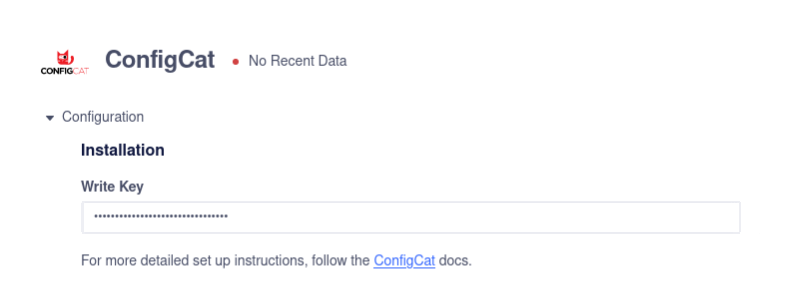
- Connect ConfigCat to Twilio Segment to track feature flag change events:
-
Open the integrations tab on the ConfigCat Dashboard.
-
Click the CONNECT button and set the
Write Key
acquired from the Twilio Segment.
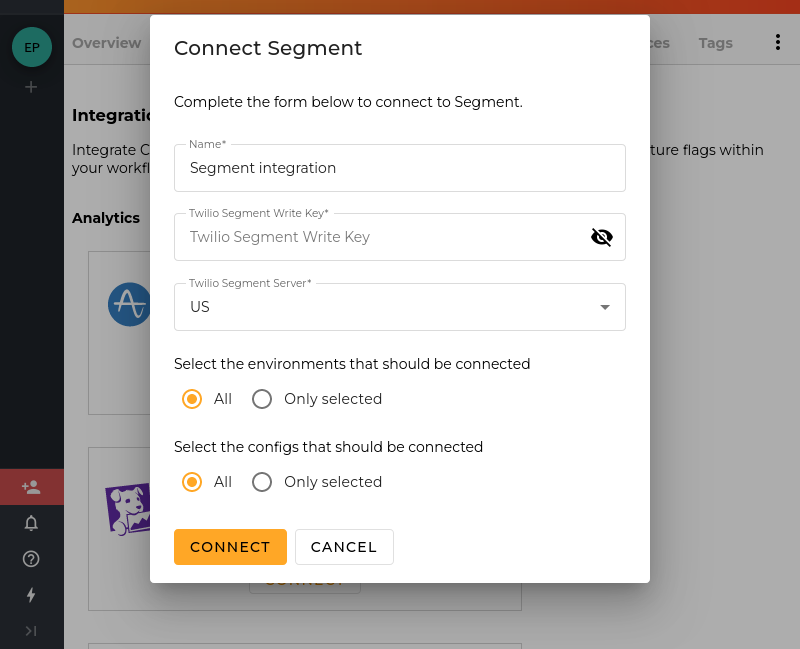
4. Connect ConfigCat with Segment in React.js
In the code below, we set up the ConfigCatProvider
to track the evaluation of feature flags. When a feature flag is evaluated, it sends the details to Segment using the Track API call.
import { ConfigCatProvider, PollingMode } from "configcat-react";
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.createRoot(document.getElementById('root')!).render(
<React.StrictMode>
<ConfigCatProvider
sdkKey="YOUR_CONFIGCAT_SDK_KEY"
pollingMode={PollingMode.AutoPoll}
options={{
pollIntervalSeconds: 5,
setupHooks: (hooks) =>
hooks.on('flagEvaluated', evaluationDetails => {
if (evaluationDetails.user) {
segmentAnalytics.identify(evaluationDetails.user.identifier, evaluationDetails.user);
}
segmentAnalytics.track('Feature Flag Evaluated', {
'feature_flag_key': evaluationDetails.key,
'value': evaluationDetails.value,
'variation_id': evaluationDetails.variationId,
'user': evaluationDetails.user
});
}),
}}>
<App />
</ConfigCatProvider>
</React.StrictMode>,
);
The Track API call is a fundamental feature in Segment, enabling you to record any actions your users perform, known as events. Each event, such as "User Signed Up" or "Button Clicked," can be enriched with properties that provide additional context, like "plan" or "accountType." This method helps you capture detailed user interactions, offering invaluable insights for analysis.
Here's a typical Track call payload in JSON format:
{
"type": "track",
"event": "User Signed Up",
"properties": {
"plan": "Pro Monthly",
"accountType": "Facebook"
}
}
And the corresponding JavaScript event in code:
analytics.track('User Account Upgraded', {
plan: 'Pro Monthly',
accountType: 'Facebook',
});
Beyond this, track calls can include fields like context
for additional data about the environment, such as the user's IP address or the page URL. This makes Track a powerful tool for collecting and analyzing user data across your platforms. You can learn more about Segment tracks here.
5. Track User Interactions in React
In my ConfigCat dashboard, I created a new feature flag named myAwesomeFeature
to enable the appropriate button and send user-click events to Twilio Segment.
import { useFeatureFlag } from 'configcat-react';
function App() {
const { value, loading } = useFeatureFlag('myAwesomeFeature', false);
if (loading) {
return <div>Loading...</div>;
}
return (
<>
{value ? (
<div>
<button onClick={() => segmentAnalytics.track('Feature Enabled')}>
Feature Enabled
</button>
</div>
) : (
<div>
<button onClick={() => segmentAnalytics.track('Feature Disabled')}>
Feature Disabled
</button>
</div>
)}
</>
);
}
export default App;
6. Connect Twilio Segment with a Destination
For this demonstration, I chose to use a single destination. You can forward your data to one or more destinations depending on your needs. In this case, I will connect to the Amplitude destination.
- Go to Amplitude's website and find the installations page, you will find a section for alternative integrations where Segment is listed.
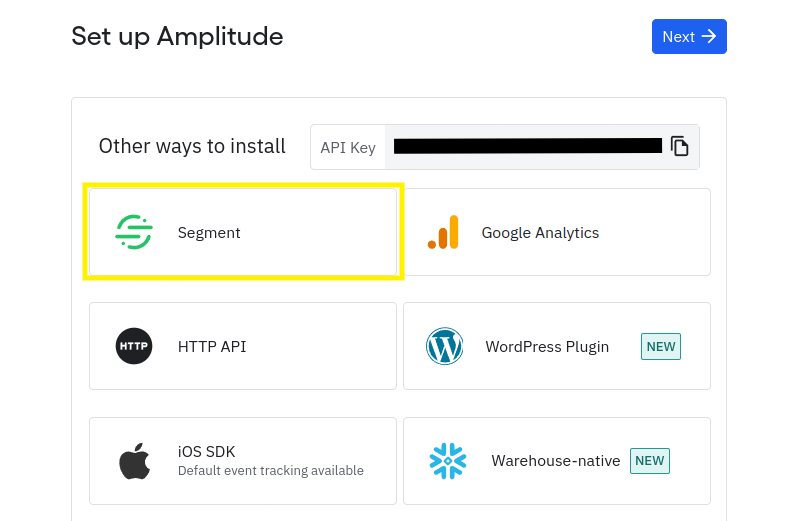
- Open the segment connections page in a new tab and add a new destination. Select Amplitude and copy over the
API key
andSecret key
. Make sure you enable the destination before saving.
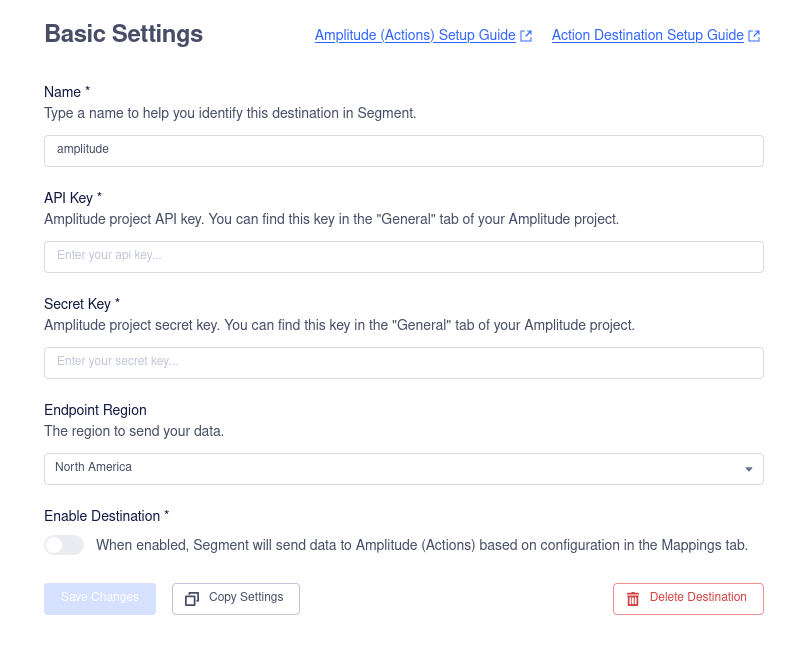
You are done! Run the React.js app and view the segment dashboard. There will be three tracked events: a button click event named either Feature Enabled
or Feature Disabled
, depending on the feature flag value; the Feature Flag Evaluated
event, showing the total number of feature flag events processed; and the number of button clicks for each.
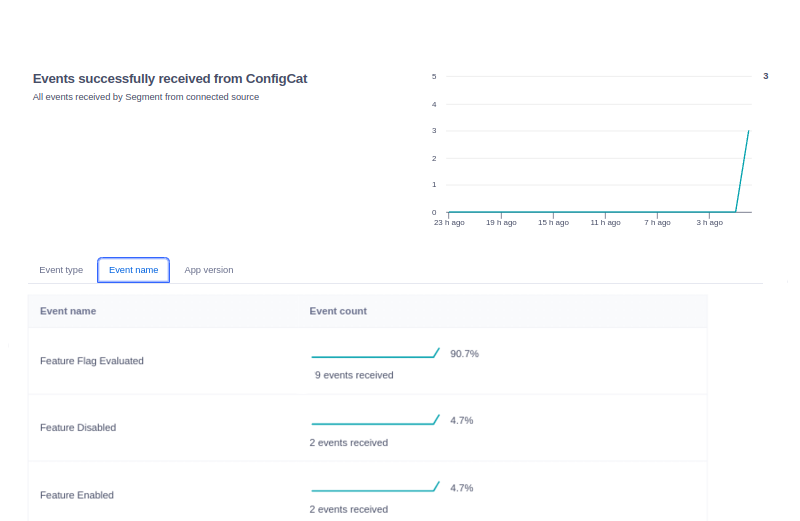
Conclusion
Integrating Twilio Segment with ConfigCat offers businesses an easy-to-use solution for managing feature flags and gaining deep insights into software changes and user interactions. This enriches the app's data gathering and adaptability for tracking changes, ensuring that businesses can obtain valuable information about their software.
For more insights on feature flags follow ConfigCat on Facebook, X, LinkedIn, and GitHub.