Using OpenFeature with ConfigCat
Let's say you've heard about the benefits of using feature flags for gradual feature rollouts, and you're ready to adopt them in your organization. The next step would be deciding whether to "build or buy."
If you choose to develop your feature flagging tool, you'll need to figure out how to make it fetch, evaluate, and manage feature flags. If you want to use a third-party tool, you might have to learn and unlearn different APIs as you search for the right one.
Challenges like these inspired the creation of OpenFeature, a CNCF (Cloud Native Computing Foundation) project that aims to standardize feature flagging.
What is OpenFeature?
OpenFeature is a specification that provides a unified API for using feature flags. Going back to our earlier scenario, this means you'd use the same code to evaluate (get the values of) feature flags when trying out feature flag platforms for your company.
How does this work?
OpenFeature provides SDKs for evaluating feature flags. Feature flag vendors (or open source contributors) then have to develop OpenFeature providers to connect their SDKs to OpenFeature SDKs. In short, OpenFeature providers act as a bridge between OpenFeature SDKs and feature flag platforms.
You don't use OpenFeature to create and manage feature flags; you only use it to evaluate them. You'll need a third-party or custom-built feature management tool to create and manage feature flags.
Benefits of OpenFeature
Here are some positive changes OpenFeature brings to feature flagging:
-
OpenFeature makes migration between feature flag platforms easier at the code level: Many feature flag platforms are compatible with OpenFeature, so switching to another vendor will involve little change in your code. With the recent release of OpenFeature's Multiproviders (currently available for JavaScript), you can use more than one feature flag platform during migration. This advantage also applies to homegrown feature flagging tools since you can create providers to make them accessible to OpenFeature.
-
OpenFeature encourages community collaboration and tool-sharing: The open-source community can collaborate and build vendor-agnostic tools around feature flags that everyone can benefit from. A good example is OpenTelemetry Hooks for OpenFeature, which you can use to attach feature flag metadata to metrics and traces.
I've said a lot about OpenFeature. Let's see how it integrates with ConfigCat, a feature management platform with first-class OpenFeature support.
Demo
For this demo, I built an AI-powered PDF extractor. The app's users can choose between different AI models for extracting data from PDFs.
I want to test newer, more powerful AI models for employees at PD-EF Corp, a fictional SaaS company, so I'll use feature flags to enable the feature for users whose emails end with @pd-efcorp.com
.
Creating the Feature Flag in ConfigCat
Let's get started.
-
Sign in to ConfigCat, or sign up if you don't have an account.
-
Create a feature flag with the following details.
- Name: Enable pro models
- Key:
enable_pro_models
- Hint: Enable pro AI models for PD-EF Corp employees
We'll add the targeting rule for PD-EF Corp employees next.
-
Click
IF +
, then clickAdd rule with user condition
.You'll see two sections: an
IF
section for configuring the condition and aTHEN
section for setting the feature flag's value based on the condition. -
In the
IF
section, selectEmail
as the comparison attribute, then selectENDS WITH ANY OF (hashed)
as the comparator.

- Enter
@pd-efcorp.com
in the field next to the comparator, then turn on the feature flag in theTHEN
section below.
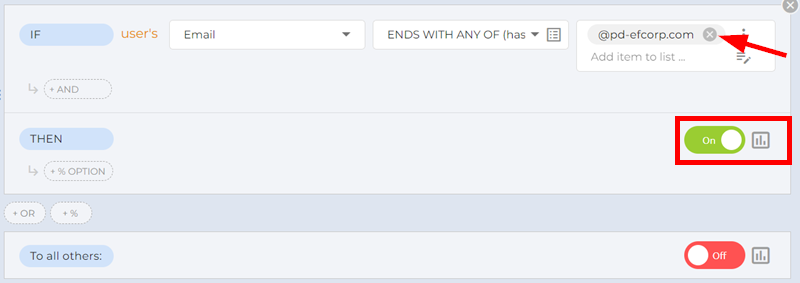
- Click
SAVE & PUBLISH CHANGES
.
Now that we've configured the feature flag, let's move on to the code.
Installing ConfigCat and OpenFeature
Prerequisites
- Node.js v18+
- Basic understanding of Node.js and Express
To use ConfigCat and OpenFeature together, we'll need to install ConfigCat's Node.js provider for OpenFeature. ConfigCat's Node SDK and OpenFeature's Server SDK will be automatically downloaded alongside the provider.
- Open your terminal and install the provider.
npm install @openfeature/config-cat-provider
- Import the provider and OpenFeature server SDK in
index.js
.
import { ConfigCatProvider } from '@openfeature/config-cat-provider';
import { OpenFeature } from '@openfeature/server-sdk';
- Create a provider with your ConfigCat SDK key. Then, set the provider and create an OpenFeature client.
// create a provider with your SDK key
const provider = ConfigCatProvider.create('YOUR-SDK-KEY');
// set the provider
OpenFeature.setProviderAndWait(provider);
// Create a client.
const client = OpenFeature.getClient();
ConfigCatProvider.create
creates a ConfigCat client with the specific SDK key. The setProviderAndWait
method sets ConfigCat as the source of feature flag values and ensures the provider is ready before we begin using it. Finally, OpenFeature.getClient
creates a client that uses the set provider to evaluate feature flags.
Using OpenFeature with ConfigCat
I have an existing /models
route for serving the list of AI models to the frontend. I'll modify its callback function to accommodate the feature flag.
app.post('/models', async (req, res) => {
// get the user's id from the database
const user = db.getUser(req.body.userId);
// fetch the models
const proModels = db.getModels('pro');
const standardModels = db.getModels('standard');
// create the evaluation context
const context = {
targetingKey: user.id,
email: user.email,
};
// evaluate the feature flag with the context
let modelsListEnabled = await client.getBooleanValue(
'models_list',
false,
context,
);
// serve the models based on the feature flag's value
if (modelsListEnabled) {
res.json(proModels);
} else {
res.json(standardModels);
}
});
I created the context
object to store the user's email and unique targeting key. Next, I evaluated the feature flag, passing in the key, default value, and context
. The provider automatically transforms context
into a ConfigCat User object, which ConfigCat will use to determine whether the user's email matches the condition I set in the targeting rule.
Finally, I added a condition to return the appropriate model based on the feature flag's value. That's all the code we'll need. Let's see how it looks.
Once I open the website and sign in with a PD-EF Corp email address, I'll see the pro AI models.
The call to the API's models/
route is implemented in the frontend. You can find the complete code on GitHub.
Why use ConfigCat with OpenFeature?
- First-class OpenFeature support: ConfigCat builds and maintains its own OpenFeature providers, which are available for most languages.
- Powerful and easy to use: Both seasoned and new feature flag users will benefit from using OpenFeature with a capable and beginner-friendly feature management platform.
- Existing ConfigCat users can benefit from an open feature-flagging ecosystem: ConfigCat users can leverage available tools in the OpenFeature ecosystem and contribute by building tools and sharing knowledge with the feature-flagging community.
Recap
Thanks for accompanying me on this short trip through OpenFeature and ConfigCat. We covered:
- What OpenFeature is.
- The benefits of OpenFeature.
- How to create a feature flag in ConfigCat.
- How to use OpenFeature with ConfigCat.
- The benefits of using OpenFeature with ConfigCat.
Next Steps
Check out OpenFeature.dev to learn more about OpenFeature and its ecosystem. You can also join the OpenFeature Slack channel to get the latest updates.
If you're not using feature flags yet, you can start with ConfigCat. ConfigCat has SDKs and OpenFeature providers for many popular programming languages and a free-forever plan suitable for small-scale feature flagging.
For more on feature flags, check out ConfigCat on Facebook, X, GitHub, and LinkedIn.