Using Feature Flags in Unreal Editor Scripts
Feature flags have equipped software developers to seamlessly roll out and roll back new features with the click of a button.
Due to their design and architecture, feature flags can be adapted and integrated into many languages and frameworks. They can be used with other technologies to enhance or add decoupled functionalities. Using them when scripting and automating your Unreal Engine editor is no exception to this.
What are feature flags?
A feature flag, or toggle, is a boolean switch that controls a feature in an application. Feature flags are commonly used within conditional statements to enable or disable features. You can control almost anything with a feature flag, including code logic, UI components, etc.
Adding feature flags to Unreal's editor scripts
Unreal Engine is one of the most advanced real-time 3D creation tools. It is popular in video game development, film, and other visual content creation. Unreal is fused with technologies like Lumen and Nanite to help you develop engaging visual content.
With the release of Unreal Engine version 4, Unreal introduced its Python API and its Python Editor Script Plugin. By combining these two, developers can script or automate parts of the Unreal Editor by using Python scripts. We can leverage this API to feature flag-specific functionalities that control the Editor.
To illustrate this, let's explore a simple example that uses Unreal's Python API and a feature flag to display a dialog in the Editor when the feature flag is enabled.
If you're looking to use ConfigCat feature flags directly in your C++ code or project blueprints, consider the ConfigCat Unreal Engine SDK. Here's a helpful guide to get you started.
Before getting started, here are the prerequisites you'll need:
Prerequisites
- Ensure your system meets Hardware and Software Specifications requirements.
- Have Unreal Engine installed.
Getting started
1. Download and install the latest version of Unreal Engine from here.
2. Create a new project by selecting GAMES, choose Third Person and click the Create button to launch the new project:
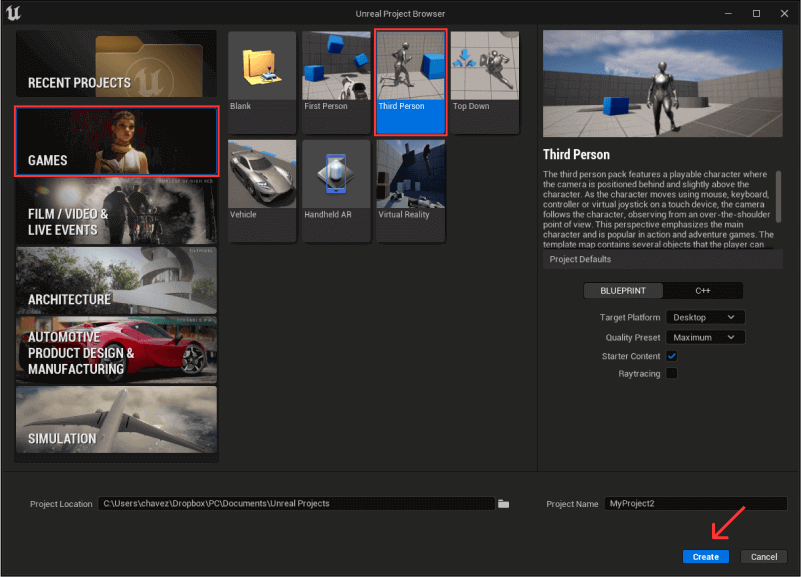
3. In the menu bar, click Edit -> Plugins then search for and enable the Python Editor Script Plugin:
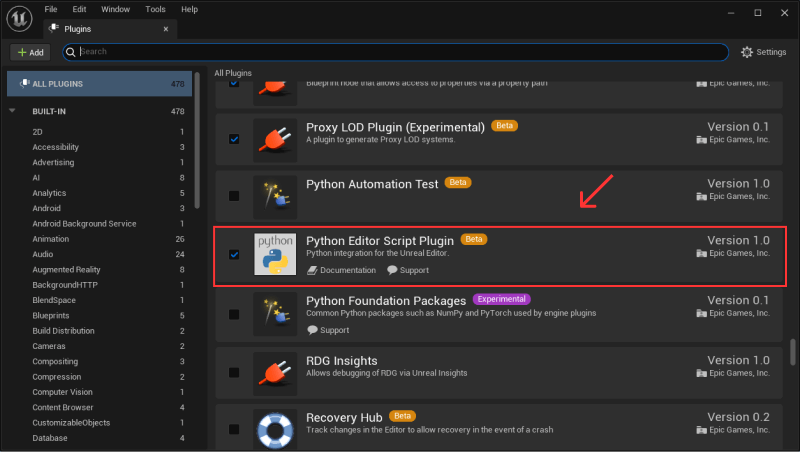
Running a script when the editor loads
According to the documentation, if the Editor detects a script file called init_unreal.py
within the Content/Python
folder in an Unreal project directory it will run that script immediately.
For example, my directory looks like this: \Documents\Unreal Projects\MyProject\Content\Python
Go ahead and create an init_unreal.py
file.
Leveraging Unreal's Python API
1. To create a dialogue and display it when the project launches, I'll use the EditorDialog
class and call the show_message
method. In the init_unreal.py
file, import unreal and add the following code below it:
import unreal
unreal.EditorDialog.show_message("Feature flag status", "This message is shown because your feature flag is enabled in ConfigCat!", unreal.AppMsgType.OK)
If you restart your project, you should be able to see this dialogue. But let's take it further and only show this message when the feature flag is enabled.
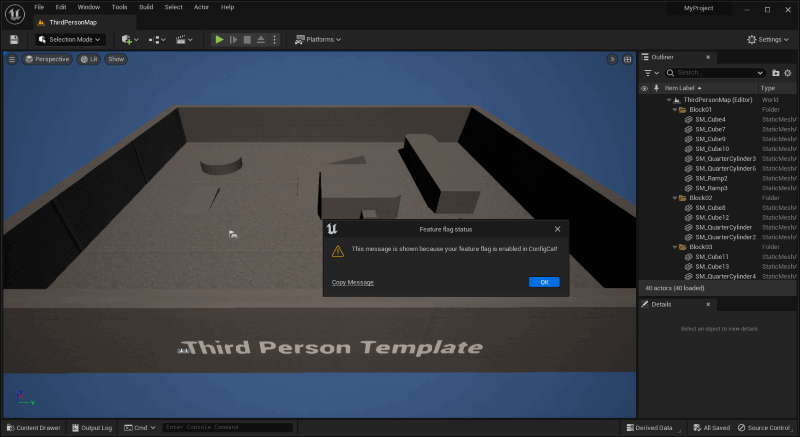
Creating a feature flag in ConfigCat
1. Login to your ConfigCat account.
2. In the dashboard, create a feature flag with the following details:
- Name: Dialog Message
- Key: dialogMessage
- Hint: Showing a dialog message in the editor
Unreal uses an embedded version of Python within its Python Editor Script Plugin, so I'll have to install ConfigCat's Python SDK client into it.
3. Find and copy the path to Unreal's Python. Mine looks like this: "C:\Program Files\Epic Games\UE_5.1\Engine\Binaries\ThirdParty\Python3\Win64\python.exe"
4. Open a command prompt, paste the path you copied above, then append configcat-client
to it and press Enter
:
"PATH-TO-UNREAL-PYTHON" -m pip install configcat-client
This will install the required functionality to establish a connection to ConfigCat to check the status of the feature flag.
5. In the init_unreal.py
file import the ConfigCat client package and create the client with your SDK key:
import configcatclient
configcat_client = configcatclient.get('YOUR_CONFIGCAT_SDK_KEY')
Your SDK key allows the Python SDK to query your account for feature flags and their status. It is generally recommended that this key be kept secure and not uploaded to your code repository.
6. Create a variable to store the value of the feature flag. This variable returns a boolean value, which I'll use in an if statement to enable or disable the dialog.
dialogmessage = configcat_client.get_value('dialogmessage', False)
if dialogmessage:
unreal.EditorDialog.show_message("Feature flag status", "This message is shown because your feature flag is enabled in ConfigCat!", unreal.AppMsgType.OK)
The complete version of the init_unreal.py
file can be found here.
Test drive
1. Head over to the ConfigCat dashboard and turn off the feature flag.
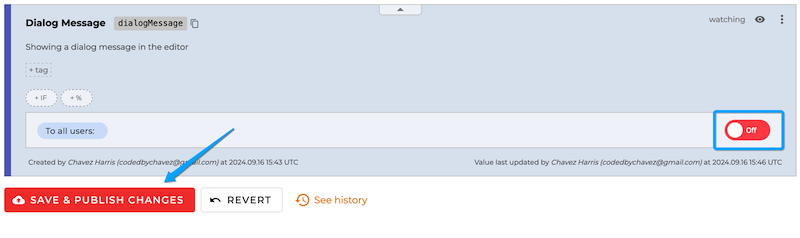
2. Relaunch the project, and you should no longer see the dialog message:
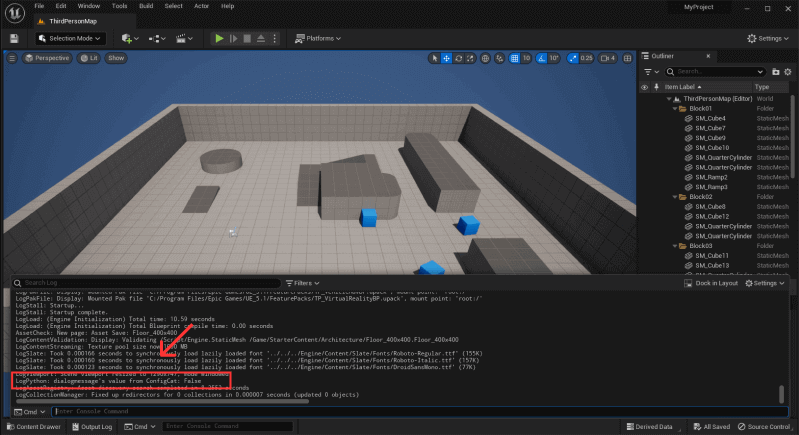
Conclusion
Because of the flexible architecture of feature flags, they can be used in different technologies to add additional functionalities. This demo only scratched the surface of what feature flags can do. They are an essential piece of the puzzle when it comes to software updates and feature rollouts.
Feature flags are quite useful for smooth canary deployments and A/B tests. They always seem to save the day and can be used in many languages and frameworks. If you haven't already, sign up for a free ConfigCat account and give feature flags a try.
Stay updated
Stay on top of the latest posts and announcements from ConfigCat on X, Facebook, LinkedIn, and GitHub.