Using Feature Flags in an Angular Application
In today's fast-paced digital landscape, users expect seamless, high-quality software experiences without interruptions. As developers, our challenge is introducing new features and updates without causing downtime. The solution? Feature flags. With feature flags, you can safely roll out new features gradually, ensuring your application remains fully operational while the development process continues.
What are feature flags?
Feature flags allow developers to toggle application features on or off without redeploying code. They are variables that can be modified remotely, eliminating the need to alter the codebase directly. This approach streamlines the process of introducing new features and personalizing user experiences. Not only are feature flags simple to use, but they also integrate seamlessly and work with many programming languages and technologies.
Using Feature Flags in Angular
In this tutorial, I'll show you how to add feature flags to an Angular application quickly. To manage and control the flags, I'll use ConfigCat feature flag service and guide you through each step.
To demonstrate, I'll create a dynamic voting page where users can vote on a randomly fetched image. In a contest scenario, you might want to stop the voting at a certain moment or toggle the feature back on later.
You can find the completed application here.
Prerequisites
- Node.js and node package manager
- Basic knowledge of HTML, CSS, and JavaScript
- A code editor installed - For example: Visual Studio Code
Create the Angular application
The first step in our journey is to install the Angular CLI. Open your terminal and run the following command:
npm install -g @angular/cli
If you already have the Angular CLI installed on your machine, you can update it using:
ng update @angular/cli
Next, create the Angular project by using the ng
command. If prompted for more information, accept the defaults by pressing enter.
ng new votingPage
To launch the app, navigate to the project folder and run the following command. The --open
flag automatically opens the app in a new browser window.
ng serve --open
If everything works correctly, you should see the default Angular app page.
Let us set up the feature flag and add it to the application.
Creating a Feature Flag
Head over to your ConfigCat dashboard. Start by clicking the Add Feature Flag button and create a new flag with the details below.
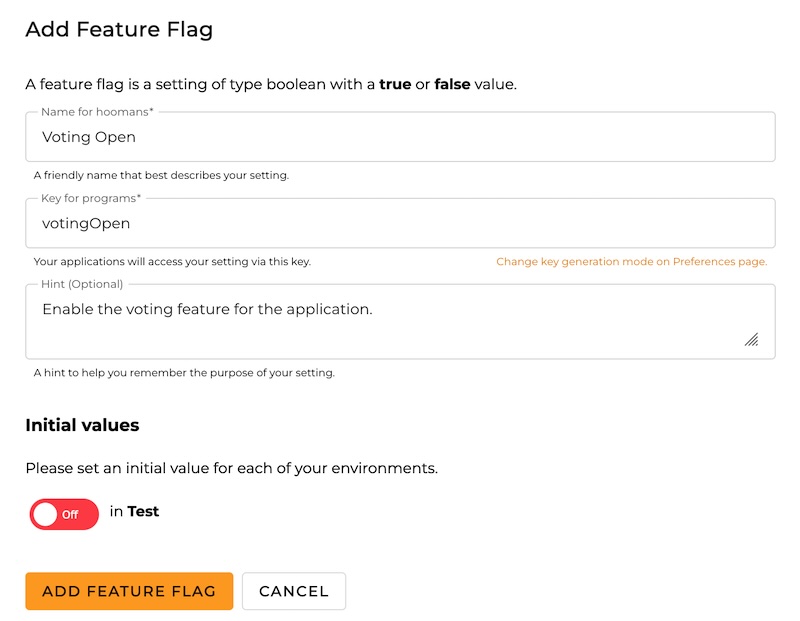
For this sample app, we will use the flag to toggle the feature for all users. However, ConfigCat provides advanced options for targeting specific user groups based on various criteria.
The final step is to locate your SDK key. Once logged in to the ConfigCat dashboard, you can find it by opening the following link: https://app.configcat.com/sdkkey.
Adding the Feature Flag to Angular
First, install the ConfigCat JavaScript SDK by executing the following command in the terminal:
npm i configcat-js
Next, import the package in the app.component.ts
file:
import * as configcat from 'configcat-js';
Next, create a local variable to store the boolean value of our feature flag. In the constructor, initialize the ConfigCat client using your SDK key:
export class AppComponent {
title: string = 'votingPage';
votingFeatureEnabled: boolean = false;
votingMode: VotingMode = 'single';
votes: number[] = [];
votingClosed: boolean = false;
countdown: number = 60;
imageUrl: string | null = null;
private countdownInterval: any;
constructor() {
const configCatClient = configcat.getClient(
'CONFIGCAT-SDK-KEY'
);
configCatClient
.getValueAsync<boolean>('votingOpen', false)
.then((value) => {
this.votingFeatureEnabled = value;
if (value) {
this.fetchRandomImage();
}
});
}
// ...
}
At this point, you will not see any changes in your browser. Let us start by displaying the initial value of our fetched feature flag. Clear the content of the app.component.html
file and add the following:
<div>Feature flag value: {{ votingFeatureEnabled }}</div>
Now that everything is connected, enhance the app.component.ts
file by adding logic to make the app more interactive.
We will add the ability to switch between single and multiple voting modes, include a 60-second voting timer, and allow users to submit a vote and see average results if the feature flag is enabled. If the flag is off, a message will show that voting is disabled.
export class AppComponent implements OnDestroy {
title: string = 'votingPage';
votingFeatureEnabled: boolean = false;
votingMode: VotingMode = 'single';
votes: number[] = [];
votingClosed: boolean = false;
countdown: number = 60;
imageUrl: string | null = null;
private countdownInterval: any;
constructor() {
const configCatClient = configcat.getClient('YOUR-SDK-KEY');
configCatClient
.getValueAsync<boolean>('votingOpen', false)
.then((value) => {
this.votingFeatureEnabled = value;
if (value) {
this.fetchRandomImage();
}
});
this.startCountdown(); // starts the 60 second countdown
}
// ...
}
You can see the complete code that contains the definitions of all functions like fetchRandomImage()
and startCountdown()
here.
In the app.component.html
file, when our feature is enabled, and the voting session is still active, we will display a form containing a countdown timer, a number input, a submit button, and the current vote average.
<div class="voting-form">
<h3>Time left: {{ countdown }} seconds</h3>
<img *ngIf="imageUrl" [src]="imageUrl" alt="Random" class="voting-image" />
<label for="number">Enter a value from <b>1 - 10</b></label>
<input name="number" type="number" #voteInput min="1" max="10" placeholder="Enter your vote" class="vote-input"
[disabled]="votingClosed" />
<button class="vote-button" (click)="submitVote(voteInput.valueAsNumber)"
[disabled]="votingMode === 'single' && votes.length > 0">
Send Vote!
</button>
<h3 *ngIf="votes.length > 0">Average Vote: {{ getAverageVote() }}</h3>
</div>
</div>
Now, head to app.component.css and add some CSS. This is optional, but it will give our application a nicer look and feel. Feel free to add your personal touch.
.container {
display: flex;
flex-direction: column;
align-items: center;
font-family: "Segoe UI", Tahoma, Geneva, Verdana, sans-serif;
width: 100%;
height: 100vh;
padding: 24px;
text-align: center;
background-color: #f9f9f9;
}
.voting-form {
display: flex;
align-items: center;
flex-direction: column;
gap: 16px;
padding: 32px;
width: 320px;
background-color: #ffffff;
border-radius: 12px;
box-shadow: 0 4px 12px rgba(122, 122, 122, 0.05);
margin: 24px 0;
}
/* ... */
Live Demo
Save the changes, and you should see the following. The voting feature is off because the feature flag was disabled.
Head to your ConfigCat dashboard and enable the feature flag.
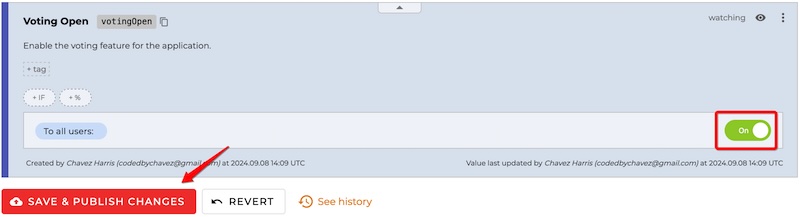
Refresh the browser page, and you should see the following result:
Conclusion
In just a few steps, you added your first feature flag to an Angular application using the ConfigCat SDK and dashboard. As I mentioned earlier, there is a lot more you can do with feature flags, like configuring user segments, running A/B tests, rolling out progressive and canary releases, and much more. ConfigCat supports many of the programming languages and frameworks you're already using.
What do you think? Pretty easy, right? ConfigCat offers a free plan for low-volume use cases, perfect for those just starting out.
You can find more information about ConfigCat on X, Facebook, LinkedIn and GitHub.